iOS开发中最经常用到的控件就是UITableView
,几乎大部分的需求都可以通过UITableView
来实现。虽然UITableViewCell
有自己的点击方法
|
|
但是我们也经常需要在UITableViewCell
中添加一些UIButton
来实现需求。因为UITableView
会使用复用机制来节省内存的使用,所以如果单纯的给cell
中的按钮添加点击事件往往会照成获取行错误的问题。
解决这个问题我们可以使用
|
|
这个方法进行点击位置坐标的转换,然后通过位置坐标,使用
|
|
这个方法来获取对应的行。
具体的使用就是这样:
- cell的创建部分:
|
|
- 点击的响应部分
|
|
实现的效果:
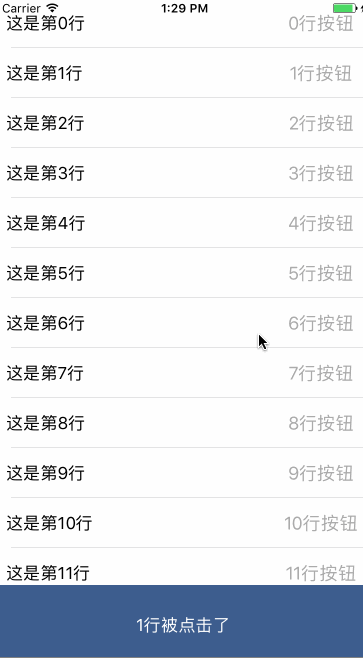
Demo可以在这里下载。